Bitget Wallet OmniConnect SDK
This section aims to guide you on how to use OmniConnect
to enable DApps
that have integrated WalletConnect
to link with Bitget Wallet
and Bitget Wallet Lite
within a Telegram Mini App. Following the content provided below will help you quickly implement this functionality.
If you are not yet familiar with how to develop a
Telegram Mini App
or useWalletConnect
to connect wallets, it is recommended to first carefully read the following documents:
Example of the Expected Result
Due to platform limitations on Telegram
, WalletConnect
currently cannot function properly within the Telegram Mini App
. If you're developing a DApp
within a Telegram Mini App
, you may encounter similar issues: whether you are trying to connect the wallet or prompt a signature, WalletConnect
cannot successfully launch Bitget Wallet
or Bitget Wallet Lite
, and either gets stuck at the launch interface or shows no response at all.
By configuring your project according to this document, you can quickly resolve these issues and enable your DApp
to use WalletConnect
properly within the Telegram Mini App
, achieving the following:
- By clicking
Connect
, a popup window will display theBitget Wallet
entry, allowing users to connect theirBitget Wallet
orBitget Wallet Lite
.
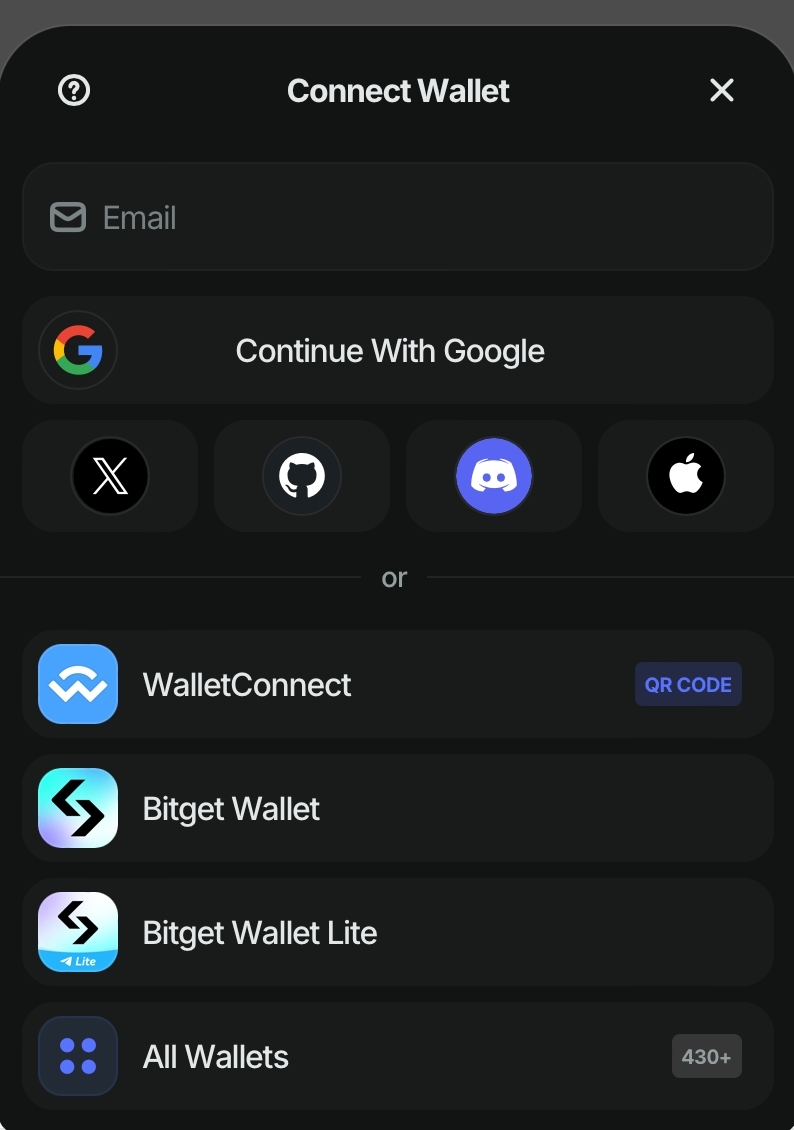
- After clicking on
Bitget Wallet
orBitget Wallet Lite
, users will be successfully redirected toBitget Wallet
for connection and signing.
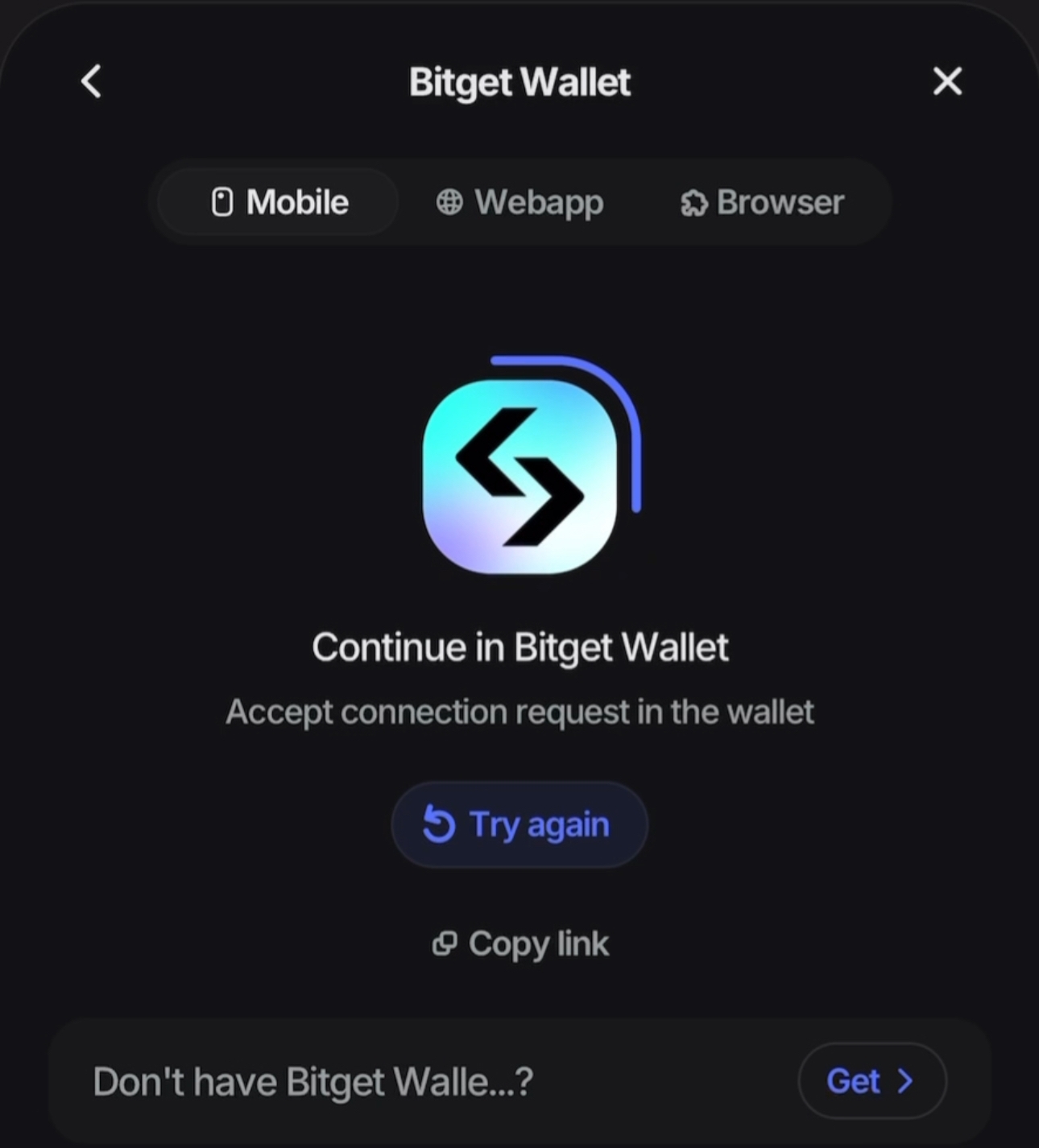
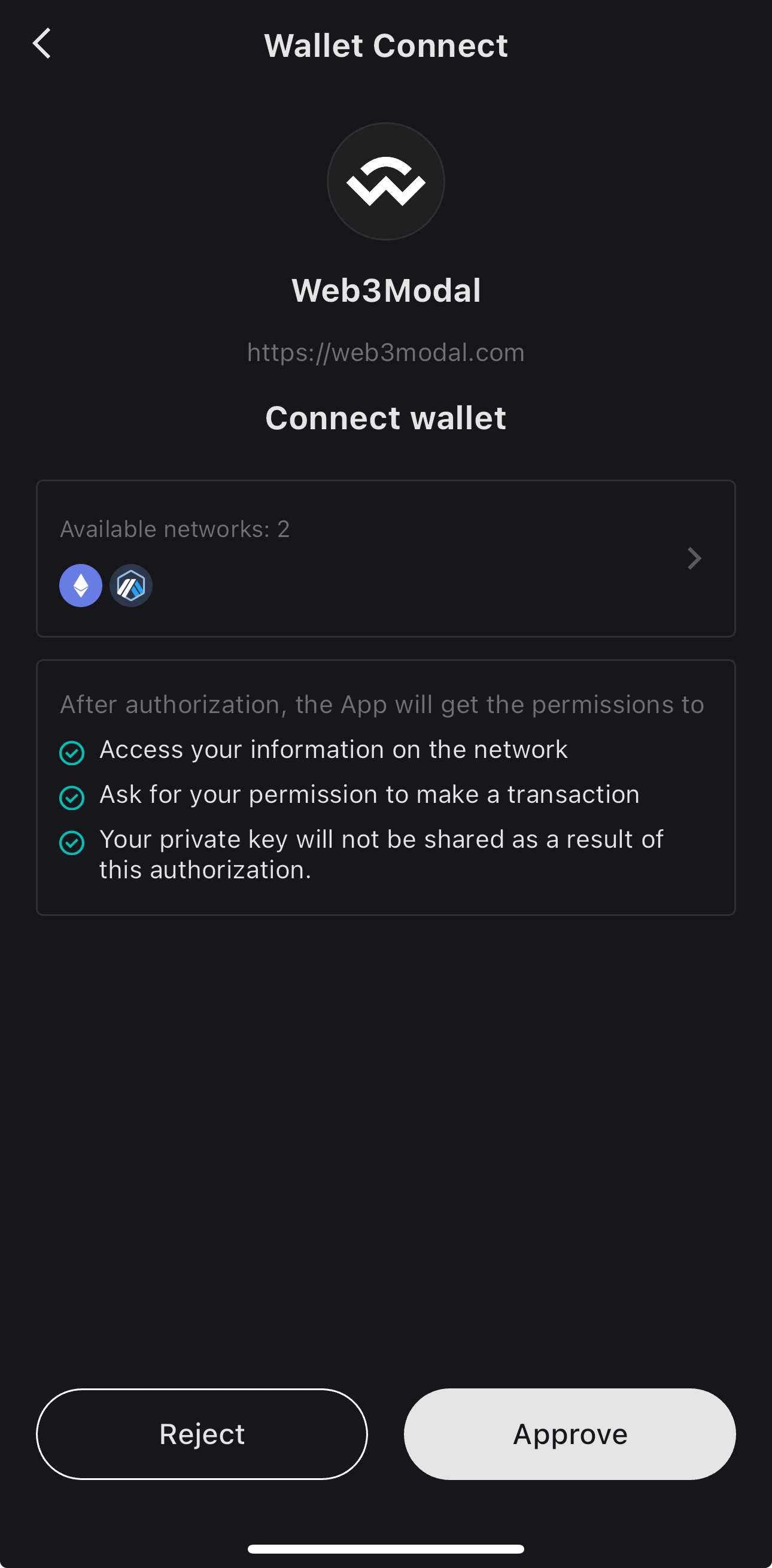
Development Process
Next, we will use the WalletConnect
official demo code to demonstrate how to achieve the above results with a few simple configurations.
1. Install Project Dependencies
npm i @bitget-wallet/omni-connect @reown/appkit-adapter-wagmi @tanstack/react-query @wagmi/core @web3modal/wagmi networks react react-dom viem wagmi
npm i @bitget-wallet/omni-connect @reown/appkit-adapter-wagmi @tanstack/react-query @wagmi/core @web3modal/wagmi networks react react-dom viem wagmi
2. DApp Initialization of WalletConnect Logic
The key point in this step is passing featuredWalletIds
into the createWeb3Modal
method. Through this configuration, Bitget Wallet
and Bitget Wallet Lite
will be displayed in the wallet selection popup of WalletConnect
.
featuredWalletIds: [
"38f5d18bd8522c244bdd70cb4a68e0e718865155811c043f052fb9f1c51de662", // Bitget Wallet project ID
"21c3a371f72f0057186082edb2ddd43566f7e908508ac3e85373c6d1966ed614", // Bitget Wallet Lite project ID
],
featuredWalletIds: [
"38f5d18bd8522c244bdd70cb4a68e0e718865155811c043f052fb9f1c51de662", // Bitget Wallet project ID
"21c3a371f72f0057186082edb2ddd43566f7e908508ac3e85373c6d1966ed614", // Bitget Wallet Lite project ID
],
// 0. Setup queryClient
const queryClient = new QueryClient();
// 1. Get projectId from https://cloud.walletconnect.com
const projectId = import.meta.env.VITE_PROJECT_ID;
if (!projectId) throw new Error("Project ID is undefined");
// 2. Create wagmiConfig
const metadata = {
name: "Web3Modal",
description: "Web3Modal Example",
url: "https://web3modal.com",
icons: ["https://avatars.githubusercontent.com/u/37784886"],
};
// Define chains
const chains = [mainnet, arbitrum] as const;
// Create the connectors
const connectors: CreateConnectorFn[] = [];
connectors.push(walletConnect({ projectId, metadata, showQrModal: false }));
connectors.push(injected({ shimDisconnect: true }));
connectors.push(
authConnector({
options: { projectId },
socials: ["google", "x", "github", "discord", "apple"], // this will create a non-custodial wallet (see https://secure.walletconnect.com/dashboard for more info)
showWallets: true,
email: true,
walletFeatures: false,
})
);
const wagmiConfig = createConfig({
chains, // Use the defined chains here
transports: {
[mainnet.id]: http(),
[arbitrum.id]: http(),
},
connectors: connectors,
});
// 3. Create modal
createWeb3Modal({
wagmiConfig,
projectId,
featuredWalletIds: ["38f5d18bd8522c244bdd70cb4a68e0e718865155811c043f052fb9f1c51de662", // Bitget Wallet project ID
"21c3a371f72f0057186082edb2ddd43566f7e908508ac3e85373c6d1966ed614", // Bitget Wallet Lite project ID
],
});
// 0. Setup queryClient
const queryClient = new QueryClient();
// 1. Get projectId from https://cloud.walletconnect.com
const projectId = import.meta.env.VITE_PROJECT_ID;
if (!projectId) throw new Error("Project ID is undefined");
// 2. Create wagmiConfig
const metadata = {
name: "Web3Modal",
description: "Web3Modal Example",
url: "https://web3modal.com",
icons: ["https://avatars.githubusercontent.com/u/37784886"],
};
// Define chains
const chains = [mainnet, arbitrum] as const;
// Create the connectors
const connectors: CreateConnectorFn[] = [];
connectors.push(walletConnect({ projectId, metadata, showQrModal: false }));
connectors.push(injected({ shimDisconnect: true }));
connectors.push(
authConnector({
options: { projectId },
socials: ["google", "x", "github", "discord", "apple"], // this will create a non-custodial wallet (see https://secure.walletconnect.com/dashboard for more info)
showWallets: true,
email: true,
walletFeatures: false,
})
);
const wagmiConfig = createConfig({
chains, // Use the defined chains here
transports: {
[mainnet.id]: http(),
[arbitrum.id]: http(),
},
connectors: connectors,
});
// 3. Create modal
createWeb3Modal({
wagmiConfig,
projectId,
featuredWalletIds: ["38f5d18bd8522c244bdd70cb4a68e0e718865155811c043f052fb9f1c51de662", // Bitget Wallet project ID
"21c3a371f72f0057186082edb2ddd43566f7e908508ac3e85373c6d1966ed614", // Bitget Wallet Lite project ID
],
});
3. DApp Initialization Logic and @bitget-wallet/omni-connect
In this step, the key is to execute the overrideWindowOpen()
method during DApp
page initialization. This will resolve the issue where WalletConnect
fails to properly launch Bitget Wallet
or Bitget Wallet Lite
within the Telegram Mini App
.
Note: The overrideWindowOpen()
method relies on the API provided by Telegram Mini App
, so it can only be executed within a Telegram Mini App
. Ensure that this method is not executed in other environments.
useEffect(() => {
const initOverrideWindowOpen = async () => {
// Check if it's a Telegram Mini App environment
const isTMA = await isTelegramEnvironment();
if (!isTMA) {
return;
}
// Execute @bitget-wallet/omni-connect initialization
overrideWindowOpen();
};
initOverrideWindowOpen();
}, []);
useEffect(() => {
const initOverrideWindowOpen = async () => {
// Check if it's a Telegram Mini App environment
const isTMA = await isTelegramEnvironment();
if (!isTMA) {
return;
}
// Execute @bitget-wallet/omni-connect initialization
overrideWindowOpen();
};
initOverrideWindowOpen();
}, []);
At this point, you have completed the configuration to use WalletConnect
to connect to Bitget Wallet
or Bitget Wallet Lite
within a Telegram Mini App
. To summarize, the two key configurations are:
1. Configure the Bitget Wallet ID within the featuredWalletIds
during the initialization of Wallet Connect
.
2. Invoke the overrideWindowOpen()
method from @bitget-wallet/omni-connect
during the initialization process.
Complete Example
import React, { useEffect, useState } from "react";
import ReactDOM from "react-dom/client";
import { createWeb3Modal } from "@web3modal/wagmi/react";
import { http, createConfig, WagmiProvider } from "wagmi";
import { mainnet, arbitrum } from "viem/chains";
import { walletConnect, injected } from "wagmi/connectors";
import type { CreateConnectorFn } from "@wagmi/core";
import { QueryClient, QueryClientProvider } from "@tanstack/react-query";
import { authConnector } from "@web3modal/wagmi";
import { isTelegramEnvironment, overrideWindowOpen } from "@bitget-wallet/omni-connect";
import "./styles.css";
// 0. Setup queryClient
const queryClient = new QueryClient();
// 1. Get projectId at https://cloud.walletconnect.com
const projectId = import.meta.env.VITE_PROJECT_ID;
if (!projectId) throw new Error("Project ID is undefined");
// 2. Create wagmiConfig
const metadata = {
name: "Web3Modal",
description: "Web3Modal Example",
url: "https://web3modal.com",
icons: ["https://avatars.githubusercontent.com/u/37784886"],
};
// Define chains
const chains = [mainnet, arbitrum] as const;
// create the connectors
const connectors: CreateConnectorFn[] = [];
connectors.push(walletConnect({ projectId, metadata, showQrModal: false }));
connectors.push(injected({ shimDisconnect: true }));
connectors.push(
authConnector({
options: { projectId },
socials: ["google", "x", "github", "discord", "apple"], // this will create a non-custodial wallet (please check https://secure.walletconnect.com/dashboard for more info)
showWallets: true,
email: true,
walletFeatures: false,
})
);
const wagmiConfig = createConfig({
chains, // Use the defined chains here
transports: {
[mainnet.id]: http(),
[arbitrum.id]: http(),
},
connectors: connectors,
});
// 3. Create modal
createWeb3Modal({
wagmiConfig,
projectId,
featuredWalletIds: [
"38f5d18bd8522c244bdd70cb4a68e0e718865155811c043f052fb9f1c51de662", // Bitget Wallet project ID
"21c3a371f72f0057186082edb2ddd43566f7e908508ac3e85373c6d1966ed614", // Bitget Wallet Lite project ID
],
});
const App = () => {
useEffect(() => {
const initOverrideWindowOpen = async () => {
// Check if the environment is a Telegram Mini App
const isTMA = await isTelegramEnvironment();
if (!isTMA) {
return;
}
// Execute the initialization method from @bitget-wallet/omni-connect
overrideWindowOpen();
};
initOverrideWindowOpen();
}, []);
return (
<React.StrictMode>
<WagmiProvider config={wagmiConfig}>
<QueryClientProvider client={queryClient}>
<div className="centered-div">
<w3m-button />
</div>
</QueryClientProvider>
</WagmiProvider>
</React.StrictMode>
);
};
ReactDOM.createRoot(document.getElementById("root") as HTMLElement).render(
<App />
);
import React, { useEffect, useState } from "react";
import ReactDOM from "react-dom/client";
import { createWeb3Modal } from "@web3modal/wagmi/react";
import { http, createConfig, WagmiProvider } from "wagmi";
import { mainnet, arbitrum } from "viem/chains";
import { walletConnect, injected } from "wagmi/connectors";
import type { CreateConnectorFn } from "@wagmi/core";
import { QueryClient, QueryClientProvider } from "@tanstack/react-query";
import { authConnector } from "@web3modal/wagmi";
import { isTelegramEnvironment, overrideWindowOpen } from "@bitget-wallet/omni-connect";
import "./styles.css";
// 0. Setup queryClient
const queryClient = new QueryClient();
// 1. Get projectId at https://cloud.walletconnect.com
const projectId = import.meta.env.VITE_PROJECT_ID;
if (!projectId) throw new Error("Project ID is undefined");
// 2. Create wagmiConfig
const metadata = {
name: "Web3Modal",
description: "Web3Modal Example",
url: "https://web3modal.com",
icons: ["https://avatars.githubusercontent.com/u/37784886"],
};
// Define chains
const chains = [mainnet, arbitrum] as const;
// create the connectors
const connectors: CreateConnectorFn[] = [];
connectors.push(walletConnect({ projectId, metadata, showQrModal: false }));
connectors.push(injected({ shimDisconnect: true }));
connectors.push(
authConnector({
options: { projectId },
socials: ["google", "x", "github", "discord", "apple"], // this will create a non-custodial wallet (please check https://secure.walletconnect.com/dashboard for more info)
showWallets: true,
email: true,
walletFeatures: false,
})
);
const wagmiConfig = createConfig({
chains, // Use the defined chains here
transports: {
[mainnet.id]: http(),
[arbitrum.id]: http(),
},
connectors: connectors,
});
// 3. Create modal
createWeb3Modal({
wagmiConfig,
projectId,
featuredWalletIds: [
"38f5d18bd8522c244bdd70cb4a68e0e718865155811c043f052fb9f1c51de662", // Bitget Wallet project ID
"21c3a371f72f0057186082edb2ddd43566f7e908508ac3e85373c6d1966ed614", // Bitget Wallet Lite project ID
],
});
const App = () => {
useEffect(() => {
const initOverrideWindowOpen = async () => {
// Check if the environment is a Telegram Mini App
const isTMA = await isTelegramEnvironment();
if (!isTMA) {
return;
}
// Execute the initialization method from @bitget-wallet/omni-connect
overrideWindowOpen();
};
initOverrideWindowOpen();
}, []);
return (
<React.StrictMode>
<WagmiProvider config={wagmiConfig}>
<QueryClientProvider client={queryClient}>
<div className="centered-div">
<w3m-button />
</div>
</QueryClientProvider>
</WagmiProvider>
</React.StrictMode>
);
};
ReactDOM.createRoot(document.getElementById("root") as HTMLElement).render(
<App />
);